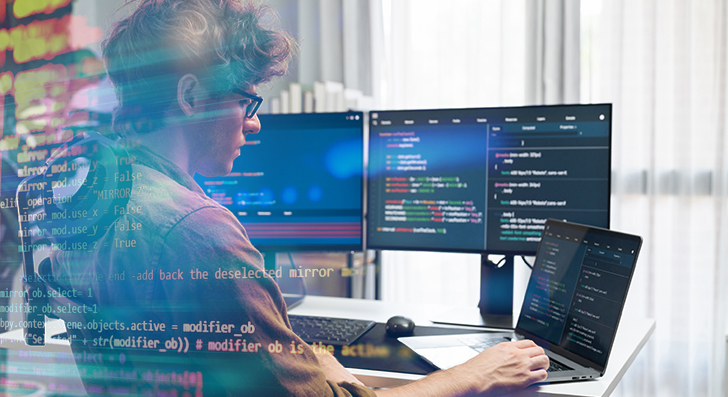
Scalability implies your software can cope with progress—a lot more users, extra knowledge, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and functional manual to help you start out by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your prepare from the start. Several purposes fall short every time they expand speedy since the first design and style can’t tackle the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where by every little thing is tightly linked. Instead, use modular design and style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself without the need of affecting The entire technique.
Also, contemplate your databases from day 1. Will it need to have to manage one million users or simply a hundred? Select the proper variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them still.
A different vital level is to stop hardcoding assumptions. Don’t produce code that only will work less than present-day disorders. Think about what would happen In case your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or celebration-pushed programs. These assistance your application cope with additional requests with no receiving overloaded.
If you Construct with scalability in mind, you're not just making ready for achievement—you might be cutting down foreseeable future head aches. A nicely-planned process is simpler to maintain, adapt, and grow. It’s improved to prepare early than to rebuild afterwards.
Use the best Database
Deciding on the suitable database is a vital Component of creating scalable applications. Not all databases are constructed a similar, and utilizing the Incorrect you can sluggish you down or even induce failures as your app grows.
Start by knowledge your info. Could it be highly structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. These are solid with relationships, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your info is a lot more flexible—like person activity logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling huge volumes of unstructured or semi-structured details and can scale horizontally far more effortlessly.
Also, look at your read and publish styles. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Will you be managing a weighty generate load? Consider databases that could tackle high compose throughput, as well as party-based information storage techniques like Apache Kafka (for momentary information streams).
It’s also wise to Consider in advance. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t need to have to switch later.
Use indexing to speed up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on database functionality when you improve.
To put it briefly, the ideal databases will depend on your application’s construction, pace requirements, and how you anticipate it to grow. Get time to pick wisely—it’ll save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, just about every smaller hold off adds up. Badly written code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start off by creating clean, easy code. Avoid repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a straightforward just one operates. Keep the features brief, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well very long to run or takes advantage of excessive memory.
Next, look at your databases queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the info you really have to have. Stay away from Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay clear of carrying out a lot of joins, especially across substantial tables.
In the event you observe the identical facts being requested time and again, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions when you can. As opposed to updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more successful.
Make sure to test with big datasets. Code and queries that perform wonderful with a hundred records may crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep your code tight, your queries lean, and use caching when needed. These actions support your application keep sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers plus more traffic. If everything goes through one server, it will quickly become a bottleneck. That’s exactly where load balancing and caching are available. Both of these equipment aid keep your app fast, secure, and scalable.
Load here balancing spreads incoming website traffic throughout a number of servers. As an alternative to a single server carrying out the many operate, the load balancer routes consumers to various servers according to availability. This suggests no solitary server will get overloaded. If 1 server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You can serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the consumer.
Caching cuts down database load, increases speed, and can make your app far more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are basic but powerful resources. Jointly, they help your app tackle a lot more people, stay quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To build scalable programs, you may need applications that let your app expand simply. That’s wherever cloud platforms and containers are available. They give you versatility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you can scale down to save money.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You could center on making your application as opposed to handling infrastructure.
Containers are An additional important tool. A container offers your application and every little thing it must run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
To put it briefly, making use of cloud and container tools signifies it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your application to mature without having restrictions, begin working with these resources early. They help save time, reduce threat, and assist you stay focused on making, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when factors go wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for users to load pages, how frequently errors happen, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems fast, often right before people even observe.
Monitoring can also be useful whenever you make changes. If you deploy a completely new attribute and see a spike in errors or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct applications in position, you stay on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for significant firms. Even small apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you may Construct applications that grow efficiently without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.